iOS Swift
This document provides the information required by Financial Institutions (FIs) with Swift iOS platform applications to prepare and pretest the implementation of Personetics widgets, and integrate them in the bank’s own application.
A sample app is initially provided. The sample app provides examples of widget implementation, and enables analyzing code integration into the FI's app. (The sample app is run separately).
Operating Systems
Supported os versions:
iOS 14.0, iOS 15.0, iOS 16.0, iOS 17.0
Deprecation policy:
- Time frame: Personetics client version 3.9 will support the iOS versions mentioned above for a period of two years from the launch date of the Personetics client version, as long as the operating system provider continues to support these versions.
- Scope: During this period, support for the Personetics client version includes updates from both Personetics client and the operating system versions.
- Upgrade implications: In case a customer upgrades their Personetics client version, this may result in changes to the supported operating system versions. Please contact your project manager for further details.
Package Description
Two compressed packages are provided:
- personetics-integration-app-swift.zip - sample app app files.
- persoKit.xcframework.zip - SDK product package . Initially vanilla, followed by the custom code.
Running the Sample App
The sample app provides visual examples of widget implementation and of server response to common API commands.
To run the sample app
- Extract the following files to an accessible location on your computer:
- personetics-integration-app-swift.zip
- persoKit.xcframework.zip
- In Xcode, from the personetics-integration-app-swift folder, open Personetics.xcworkspace with Xcode
- Drag the persoKit.xcframwork folder (from your computer), into frameworks folder.
The 'Choose options for adding these files' dialog opens.
- Verify the definitions are as illustrated below:
- 'Copy items if needed' is selected
- 'Added folders' set to 'Create folder references'
- 'Add to targets' is checked and defined to 'Personetics-integration-app'
- Click Finish. The Xcframework files are added to the Frameworks folder as illustrated below.
-
Click under your project/workspace (e.g. Personetics-Integration-app), and in the workspace, click the General tab.
-
Under Frameworks, Libraries, and Embedded Content, verify the status of the persoKitxcframework file is set as Embed & Sign.
- Click on Build Phases tab and scroll to Link Binary with Libraries, and verify persoKitxcframework file is listed.
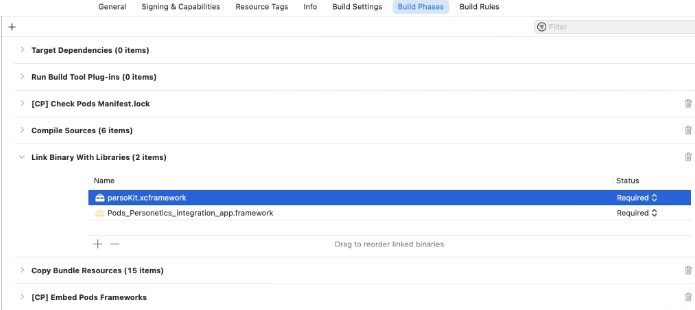
- Under Link Binary with Libraries, verify that the Status of each the following files is set to Required:
- PersoKit.xcframework
- Pods_Personetics_integration_app.framework
- Open the Configuration file, and set the key 'isPredefinedMode' to 'true'.
- (Build and) run the project. The sample app appears.
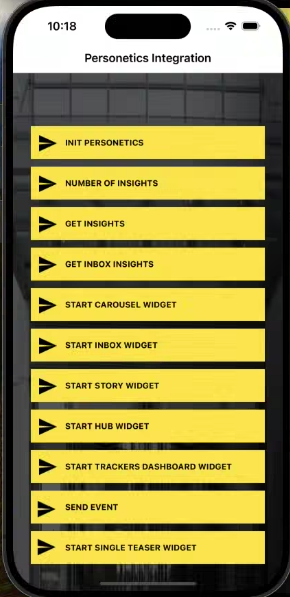
The app provides two types of elements:
- Responses to API requests. For example, Number of Insights, displays the response to Get Number of Insights.
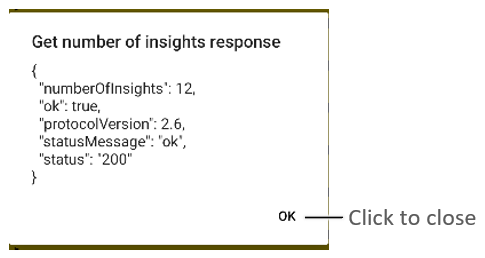
-
Widgets operations – click on the relevant widget. For example, Start Hub Widget, displays an example of hub widget implementation.
Note: The Budget and Subscription widgets can be accessed by running the Trackers Dashboard or Hub (for Budget only) widget, and clicking the Budget Tracker.
Integration
This section describes how to integrate Personetics vanilla or custom app into the bank’s application and provides explanations on the relevant files, available parameters, and code implementation. The process is the same for both vanilla and sample code.
To integrate Personetics code into the bank’s application
- Integrate the .xcframework into the iOS Swift project
- Configure Event Handlers
- Call startWidget
Note: Functions receive ViewController response (from startWidget). The bank can determine how to display the response (push, add, etc.).
Integrating .xcframework into the Bank's App
To Integrate the .xcframework into the iOS Swift project
- Extract the persoKit.xcframework.zip file into an accessible location on your computer. The folder is extracted.
- In Xcode, define a frameworks folder in the appropriate location in you project _main directory
- Drag the extracted persoKit.xcframwork file (from your computer) to the Framworks folder.
- The 'Choose options for adding these files' opens. Verify the definitions are as illustrated below:
- 'Copy items if needed' is selected
- 'Added folders' set to 'Create folder references'
- 'Add to targets' is checked and defined to your bank-project-name.
- Click Finish. Xcframework is added to your project Frameworks folder as illustrated below.
- Click under your project/workspace, and in the workspace, click the General tab.
- Under Frameworks, Libraries, and Embedded Content, verify the status of the persoKitxcframework file is set as Embed & Sign.
- Click on Build Phases tab and scroll to Link binary with libraries, and verify persoKitxcframework file is listed.
- Verify that the Status of PersoKit.xcframework file is set to Required:
Configure Event Handlers
Create event handlers, referring to the following:
- BankPersoneticsDelegate.swift – file in SDK containing examples of function implementation.
- Brief descriptions of relevant delegate functions and their syntax
- Detailed descriptions of event handlers - Widget API
Note the following:
Personetics iOS Swift is implemented using the Delegate control structure:
- Control Delegate – controls all the actions and defines the protocol structure. It contains all the required commands, data and other information required for command delegation implementation.
- View Controller – contains the conditions for carrying out the commands listed and detailed in the Control Delegate view and reference to the classes detailed in the Controller view.
To assist the customer in analysis, two files are provided:
- BankPersoneticsDelegate.swift - Example of event handlers functionality implementation for the Personetics iOS Swift Vanilla
- ViewController.swift - Located under Personetics-integration-app. Illustrates functionality of the Personetics SDK. This includes backend API example, and startWidget implementation (i.e. how to initiate widgets).
Note: The two code files include a range of examples. For all available functionality, refer to the Widget API document.
Call startWidget
To call startWidget:
- Create the Dictionary
- Add the keys to the Dictionary
- Use the startWidget response object on the view controller
Refer to the ViewController.swift
file, and the example below.
startWidget Implementation Example
Following are examples for startWidget implementation including: creating dictionary, bridge to delegate, sending dictionary to widget.
- Initiate personeticsInfo and all needed objects (Create dictionary).
let personeticsInfo = NSMutableDictionary.init() let configurations = NSMutableDictionary.init() let params = NSMutableDictionary.init() let assets = NSMutableDictionary.init() let internationalization = NSMutableDictionary.init() let theme = NSMutableDictionary.init()
- Create personeticsBridge Create delegate object
let personeticsBridge = BankPersoneticsDelegate.init()
- Add keys to the dictionaries. Set all the relevant properties to personeticsInfo.
Following are examples of some keys. Refer to the Key List and Examples, for a description of all available keys, required and optional according to your implementation.
params.setValue(<userName>, forKey: Personetics.PDBuserId)
params.setValue(<insightId>, forKey: Personetics.PDBinsightId)
params.setValue(<payload>, forKey: Personetics.PDBpayload)
configurations.setObject(<widgetType>, forKey: Personetics.PDBWidgetType)
configurations.setObject("ios", forKey: Personetics.PDBDeviceType)
configurations.setObject(<selectorString>, forKey: Personetics.PDBSelectorString)
configurations.setObject(params, forKey: Personetics.PDBparams)`
assets.setObject(<boolean>, forKey: Personetics.PDBuseRemoteAssets)
assets.setObject(<remoteUrl>, forKey: Personetics.PDBbaseUrl)`
internationalization.setObject(<Language>, forKey: Personetics.PDBlanguage)
theme.setValue(<boolean>, forKey: Personetics.PDBdarkMode)
personeticsInfo.setValue(personeticsBridge, forKey: Personetics.PDBpDelegate)
personeticsInfo.setValue(configurations, forKey: "configurations")
personeticsInfo.setValue(assets, forKey: "assets")`
personeticsInfo.setValue(internationalization, forKey: "internationalization")
personeticsInfo.setValue(theme, forKey: "theme")
personeticsInfo.setValue(personeticsBridge, forKey:Personetics.PDBpDelegate)
- Initiate personetics object (Create a Personetics object)
let personetics = Personetics.init()
- Link class Personetics to delegate
personeticsBridge.personetics = Personetics
- Send
personeticsInfo
previously created to startWidget
Call Personetics startWidget “startWidget” API function
let personeticsController = personetics.startWidget(personeticsInfo: personeticsInfo) as? UIViewController
- Use the object that is returned by StartWidget
self.navigationController?.pushViewController(personeticsController, animated: true)
Event Handlers
This section lists all the available functions for the creation of the Class Delegate Protocol file. Examples of some implementations are provided in the BankPersoneticsDelegate.swift file.
Function Name | Description |
---|---|
func navigateToPage(params: NSDictionary) | Values can optionally be assigned (function must be included). Description: Relevant if Call To Action is activated. Personetics invokes this function when user clicked a certain button that requires the bank action. The bank should use the provided information to navigate to the relevant page. |
func personeticsEvent(params: NSDictionary) | Value definitions required for specific products. Description: Triggered by events of on incidents captured on the web. Example: Event ‘teaserClick’ is generated when the end-user clicks on a teaser. In this case, the bank should implement (write code) for relevant behavior, where the default behavior implementation opens the story widget. For a full list of events, refer to Widget Client API doc, Delegate Callbacks section, WidgetEvent, Event Types. Note: There are various event types. Be sure to check what is the event type and act according to the business use-case. |
func sendRequestToPServer(params: String, onSuccess: @escaping (Any?) ->Void, onError: @escaping (String?) -> Void) | Mandatory – Personetics does not support independent HTTP calls. All HTTP calls should be made by the bank secured channel using the bank application code. Personetics calls this function when an HTTP request should be made to the server. The function sends two properties: params: Should be sent as the body of the request. onSuccess: Should be passed back with the response. onFailure: Should be passed back with the response. |
func sendRequestToEnrichServer(params: String, onSuccess: @escaping (Any?) -> Void, onError: @escaping (String?) -> Void) | Mandatory – Personetics does not support independent HTTP calls. All HTTP calls should be made by the bank secured channel using the bank application code. Personetics calls this function when an HTTP request should be made to the server. The function sends two properties: params: Should be sent as the body of the request. onSuccess: Should be passed back with the response. onFailure: Should be passed back with the response. |
func sendRequestToServer(params: String, serverUrl: String, onSuccess: @escaping (Any?) -> Void, onError: @escaping (String?) -> Void) | Mandatory. Personetics supports two types of services: Pserver and Enrich server. Following a Personetics call to one of the two services (Pserver/Enrich), this function will be automatically called when an HTTP request should be made to the services. The bank should send an HTTP request. The body of that request is the same body passed by this function. When getting a server response, the bank should call “onSuccess” with the server response. In general, communication with the server – Post parameters to server with request body (this is a delegate function). In addition to the parameters received from RequestToServer, the URL parameter is also received from the Pserver/EnrichServer functions. |
func sessionError(params: NSDictionary) | Optional. Do not have to assign values to function BUT function must be included in the ‘configuration file’ Personetics will call this function in case of an error. |
func sessionEnded(params: NSDictionary) | Optional. Relevant only if Personetics’ widgets have a “Close” button according to the design Personetics will call this function when the story “Close” button is clicked. Default behavior: The bank should close the controller\View. |
Key List and Examples
Key | Example |
---|---|
Personetics.PDBWidgetType | configurations.setObject("inbox", forKey: Personetics.PDBWidgetType as NSCopying) |
Personetics.PDBDeviceType | configurations.setObject("ios", forKey: Personetics.PDBDeviceType as NSCopying) |
Personetics.PDBlanguage | internationalization.setObject("en", forKey: Personetics.PDBlanguage as NSCopying) |
Personetics.PDBuserId | params.setValue(constants.userName, forKey: Personetics.PDBuserId) |
Personetics.PDBpayload | params.setValue(jsonResponse, forKey: Personetics.PDBpayload) |
Personetics.PDBSelectorString | configurations.setObject("#root", forKey: Personetics.PDBSelectorString as NSCopying) |
Personetics.PDBprotocolVersion | personeticsInfo.setValue("2.6", forKey: Personetics.PDBprotocolVersion) |
Personetics.PDBwebViewTag | personeticsInfo.setValue(1000, forKey: Personetics.PDBwebViewTag) |
Personetics.PDBpDelegate | personeticsInfo.setValue(personeticsBridge, forKey: Personetics.PDBpDelegate |
Personetics.PDBctxId | configurations.setValue("dashboard", forKey: Personetics.PDBctxId) |
Personetics.PDBWidgetHeight | personeticsInfo.setValue(400, forKey: Personetics.PDBWidgetHeight) |
Personetics.PDBWidgetWidth | personeticsInfo.setValue(300, forKey: Personetics.PDBWidgetWidth) |
Personetics.PDBinsightId | configurations.setValue("\<self.insightId>", forKey: Personetics.PDBinsightId) |
Personetics.PDBUseCaseId | configurations.setValue("\<self.useCaseId>", forKey: Personetics.PDBUseCaseId) |
Personetics.PDBinstanceId | params.setValue("\<self.instanceId>", forKey: Personetics.PDBinstanceId) |
Updated 2 months ago