React Native
This document provides the information required by Financial Institutions (FIs) with React Native platform applications to prepare and pretest the implementation of Personetics widgets, and integrate them in the bank’s own application.
A sample app is initially provided. The sample app provides examples of widget implementation, and enables analyzing code integration into the FI's app. (The sample app is run separately).
Target react-native 0.71.8
Package Description
Initially, two packages are provided:
- sample-app.zip - contains the sample application files
- personetics_react-native-vx.x.x.tgz - Vanilla SDK product files, for React Native.
Running the Sample App
The sample app provides visual examples of widget implementation and of server response to common API commands.
To run the sample app
-
Create a folder in an accessible location on your computer, and copy the sample-app.zip and *tgz file to the folder.
-
Extract sample-app.zip in the folder.
-
Open the folder files in your code editor (e.g. VS Code).
-
In your code editor, open a new terminal and run:
yarn
on the project terminal. The relevant libraries are installed. -
In the package.json file, under "dependencies", verify the dependency "personetics/react-native" is defined as follows:
"personetics/react-native": "file:./personetics-react-nativev1.01.tgz"
- Change to the relevant directory. For example, for iOS:
cd ios
- Run:
pod install
. - Return to the main directory: ../
- Run yarn for the relevant platform:
o For iOS –yarn ios
o For Android –yarn android
- The Sample App appears. Verify that Current Mode (top-right corner), is set to Predefined.
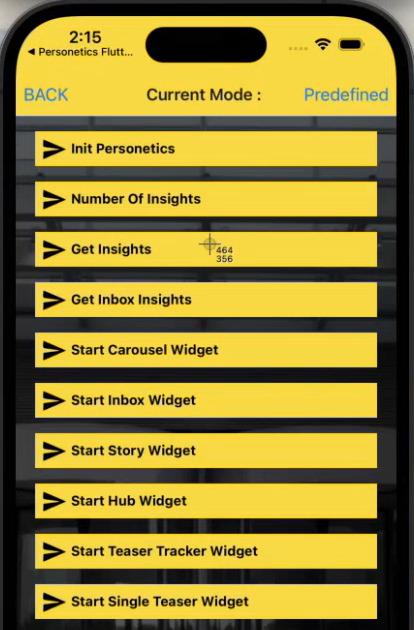
The app provides two types of elements:
- Responses to API requests. For example, Number of Insights, displays the response to Get Number of Insights.
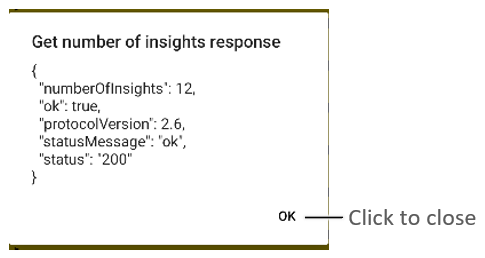
- Widgets operations – click on the relevant widget. For example, Start Hub Widget, displays an example of hub widget implementation. (To go back to the main screen, click the 'Back' control button in the device emulation pane.)
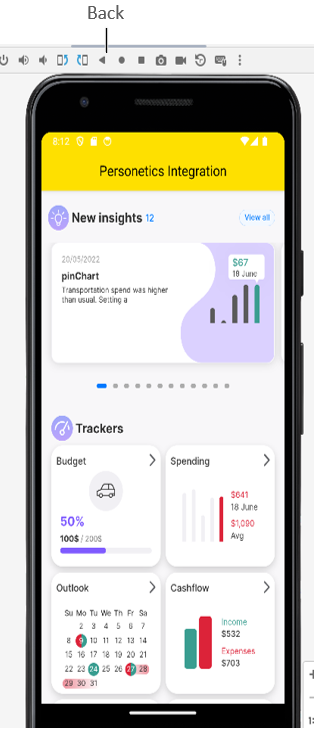
Note: The Budget and Subscription widgets can be accessed by running the Dashboard Trackers or Hub widget (Budget only), and clicking the relevant tracker.
SDK Integration Process
To integrate the SDK into your project
- Add the react native*.tgz file.
- Create engage.controller
- Configure class delegate
- Call startWidget
Add the React Native *.tgz file
Via yarn, add the file rn-engage_.tgz using the command:
yarn add <path to _tgz file> /personetics-react-native-vx.x.x.tgz
Create the Engage Controller
- Import the engage package:
import * as Engage from '@personetics/react-native';
- Create the component which returns a controller of the engage package.
return (
\<Engage.Controller
/>
);\`
- When calling the engage.controller component, send the relevant function call (delegate function for callback) and the startWidget data (initialConfig).
Example:
return (
<Engage.Controller
initialConfig={initialConfig}
setInitialConfig = {setInitialConfig}
sendRequestToPServer={sendRequestToPServer}
personeticsEvent={personeticsEvent}
navigateToPage={navigateToPage}
sessionError={sessionError}
sessionEnded={sessionEnded}
/>
);
Configure Class Delegate
Create protocol with class Delegate and implement the Delegate functions provided by Personetics. Refer to the following:
- Code file BankPersoneticsDelegate.js for examples of function implementation.
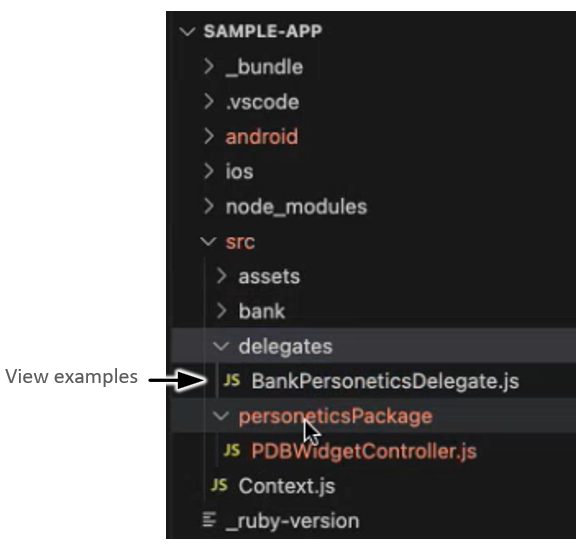
- List of the Class Delegate Functions
- Widget API document
Call startWidget
The startWidget function is used to render any Personetics widget within the bank page. The function may be accompanied by an additional payload. Refer to the Widget API document for a full description of the startWidget function and the relevant parameters.
Call StartWidget by doing the following:
Code example
import * as Engage from '@personetics/react-native';
import * as engage from '../delegates/BankPersoneticsDelegate';
In order to implement call startWidget, send the relevant data to the engage.controller component that was created earlier as the initialConfig.
Example:
<Engage.Controller initialConfig={initialConfig} />
Note: These are the functions the bank wrote in the delegateController and references must be made to these functions.
Example:
advanceConfig = {
assets: {
useRemoteAssets: USE_REMOTE_ASSETS,
baseUrl: BASE_ASSETS_URL,
},
configurations: {
protocolVersion: PROTOCOL_VERSION,
reportingEventsEnabled: false,
widgetType: 'carousel',
initialWidgetType: '',
deviceType: DEVICE,
ctxId: DASHBOARD,
selectorString: '#root',
params: {
userId: USER_NAME,
insightId: '',
persoEBMode: false,
payload: {},
},
},
internationalization: {
language: DEFAULT_LANG,
},
theme: {
darkMode: DARK_MODE,
},
};
Class Delegate Functions
The following table lists all the available functions for the creation of the Class Delegate Protocol file. Examples of some implementations are provided in the BankPersoneticsDelegate.js file.
Function Name | Description |
---|---|
const navigateToPage = (webView, obj, context) => {} | Relevant if Call To Action is activated. Personetics invokes this function when user clicks a certain button that requires the bank action. The bank should use the provided information to navigate to the relevant page. Note: the function must be included - with or without values. |
const personeticsEvent = ( webView,obj,context,setCanGoBackCallback ) => {} | Triggered by events of on incidents captured on the web. For example: the event ‘teaserClick’ is generated when the end-user clicks on a teaser. In this case, the bank should implement (write code) for the desired behavior, where the default behavior opens the story widget. For a full list of events, refer to Widget Client API doc, Delegate Callbacks section, WidgetEvent, Event Types. Value definitions required for specific products. Note: There are various event types. Note the event type and set behavior according to the business use-case. |
const sendRequestToPServer = (webView, requestBodyParams, context) => {} | Personetics calls this function when an HTTP request should be made to the server. The function sends the following properties: • WebView – app webView • requestBodyParams – Should be sent as the body of the request • context – app context Mandatory – Personetics does not support independent HTTP calls. All HTTP calls should be made by the bank secured channel using the bank application code. |
const sessionError = (webView, obj) => {}; | Optional. Do not have to assign values to function BUT function must be included in the ‘configuration file’ Personetics will call this function in case of an error. |
const sessionEnded = ( config,webView,obj,canGoBack,setCanGoBack, onClose) => {} | Optional. Relevant only if Personetics’ widgets have a “Close” button according to the design Personetics will call this function when the story “Close” button is clicked. Default behavior: The bank should close the controller\View. canGoBack and setCanGoBack – responsible for the app’s navigation |
injectPersoneticsWebview = ( fromCall,webview,data,requestId,) => { | Sends information to the webView. |
toggleModal event
This is an additional option.
Event Type: toggleModal
Description
- Allows overriding the device ‘back’ button operation when the Transaction modal is open.
- Closes only the modal, when modal is open and user clicks on the device ‘back’ button.
Event values:
True = Transaction modal was opened
False = Transaction modal was closed
Example
- Initialization of Native Event Emitter, and initial modal state.
(The NativeEventEmitter should be forwarded to the webView controller.)Callback operation that is initiated when the end-user clicks the device ‘Back’ button.const handlePersoMoadlEvent = Platform.OS === 'ios' ? null : new NativeEventEmitter(); const [isPersoModalOpen, setIsPersoModalOpen] = useState(false);
The callback indicates the status of the modal, and initiates the following responses: -
- If the modal is open, the ‘closePersoModal’ event is sent to the webView to close the modal
- If the modal is closed then the default behavior of the ‘Back’ button is applied.
const handleBackPress = useCallback(() => { if (isPersoModalOpen){ handlePersoMoadlEvent.emit('closePersoModal',\[]); } else{ setInitialConfig(''); } return true; }, [setInitialConfig,isPersoModalOpen]);
Updated 3 months ago